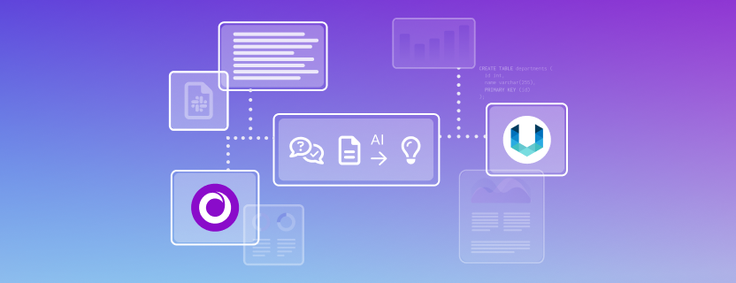
Learn how to build a Retrieval Augmented Generation (RAG) system for specific Q+A retrieval using Unstructured and SingleStore.
As ML engineers and data scientists, we're no strangers to the vastness of data and its challenges. Among the myriad solutions, Retrieval Augmented Generation (RAG) stands out as a beacon, promising enhanced information retrieval and generation capabilities. Today, we're diving deep into how we can harness the power of RAG using Slack data to answer technical questions about Unstructured open-source tools. We’ll use SingleStoreDB, a vector-capable SQL database to store the data. You can follow the codes on Google Colab.
What is Retrieval Augmented Generation (RAG)?
RAG presents a unique opportunity, allowing us to tap into vast datasets (like Slack channels) to fetch and generate information on the fly. Whether it's onboarding, technical troubleshooting or project updates, RAG based on Slack data can be a game-changer — providing instant, detailed responses.
At its core, RAG is a two-step process:
- Retrieval. This phase acts like a search engine, delving into vast datasets to retrieve relevant snippets based on a query.
- Augmentation. Once the relevant data is fetched, a language model like GPT-4 or PaLM-2, enhances and refines the response to ensure accuracy and coherence.
Laying the foundation
This comprehensive guide introduces readers to the intricacies of building a RAG system tailored for extracting and generating insights from Slack conversations. Central to this endeavor is a robust technical arsenal comprising:
- Unstructured tools. Connect and transform Slack data into a format primed for vector databases and Large Language Models (LLMs).
- SingleStoreDB. A performant, vector-capable SQL storage solution where all Slack conversations are systematically organized and indexed.
- LangChain. An LLM orchestrator designed to enhance the efficiency of chained language models and other components.
- OpenAI's GPT. An LLM with human-level text understanding and generation.
You'll also need:
- OpenAI API key
- Slack token
Unstructured API key
Singlestore Helios account
Code walkthrough
As we progress, the guide elucidates the foundational steps pivotal for a successful RAG system deployment:
- Readers are guided through a structured roadmap, from the installation of requisite libraries to the secure configuration of APIs.
- The subsequent phases spotlight data ingestion, emphasizing the significance of fetching and preparing Slack data for optimal RAG performance.
- The guide then culminates in a deep dive into the core of RAG, the retrieval and augmentation phases. Here, readers gain insights into the mechanics of document splitting, data retrieval, embedding generation and the nuances of crafting refined, precise responses using state-of-the-art models.
Installing and importing libraries
Before initiating our journey, we must first install several essential packages. These include openai
for interfacing with OpenAI's GPT models, langchain
for potentially chaining language models, tiktoken
to count tokens in strings without API calls, unstructured
libraries tailored for handling unstructured data (with specific functionalities for Slack) and singlestoredb
to interact seamlessly with SingleStoreDB.
!pip install openai langchain tiktoken
!pip install "unstructured[slack]" unstructured_inference
!pip install singlestoredb
You also need to configure the OpenAI API key and SingleStoreDB connection.
import os
import openai
openai.api_key = os.environ["OPENAI_API_KEY"]
os.environ["SINGLESTOREDB_URL"] = "<REPLACE SINGLESTORE_URL>"
Ingesting Slack data using the Unstructured.io connector
In this step, we focus on extracting data from a Slack channel using the unstructured ingest
command. This command, tailored for pulling Unstructured data, requires a specific Slack channel ID and token. The retrieved data is then saved locally in designated directories.
To utilize Unstructured's hosted API during this process, the --partition-by-api
flag is essential, and the provision of an API key is mandatory. Upon execution, the Slack data is prepared and primed for subsequent steps.
import subprocess
command = [
"unstructured-ingest",
"slack",
"--channels", "<REPLACE CHANNEL URL>",
"--token", "<REPLACE SLACK TOKEN>",
"--download-dir", "slack-ingest-download",
"--structured-output-dir", "slack-ingest-output",
"--partition-by-api",
"--api-key", "<REPLACE UNSTRUCTURED API KEY>",
"--reprocess", "--preserve-downloads"
]
# Run the command
process = subprocess.Popen(command, stdout=subprocess.PIPE)
output, error = process.communicate()
# Print output
if process.returncode == 0:
print('Command executed successfully. Output:')
print(output.decode())
else:
print('Command failed. Error:')
print(error.decode())
Load and process the data for chunking and embedding with LangChain
The ingested Slack data is loaded using LangChain’s TextLoader
object, preparing the content for subsequent processing in the notebook. Following this step, we need to manage the volume of ingested data by dividing it into smaller segments. For this, the CharacterTextSplitter
utility segments extensive texts based on a predetermined character count, optimizing the processing in the ensuing stages. The last step in data processing emphasizes the significance of embeddings (dense vector representations of texts) in information retrieval systems. Utilizing the OpenAIEmbeddings
class, embeddings are crafted using OpenAI models, setting the stage for future storage and swift data retrieval.
# Load data to LangChain object
loader = TextLoader("slack-ingest-download/C044N0YV08G.txt")
documents = loader.load()
# Split text into smaller chunk size
text_splitter = CharacterTextSplitter(chunk_size=1000, chunk_overlap=100)
texts = text_splitter.split_documents(documents)
# Embed texts using OpenAI model
embeddings = OpenAIEmbeddings()
Storing data into SingleStoreDB
The processed text chunks and their associated embeddings are stored in SingleStoreDB for swift retrieval later. This is facilitated by the SingleStoreDB.from_documents()
function from LangChain. By using this method, the system is optimized to fetch pertinent text chunks using their embeddings when queried promptly.
docsearch = SingleStoreDB.from_documents(texts,
embeddings,
table_name = "slack_data")
Query the data
In the final step, the RetrievalQA
model s tailored using a predefined prompt template. This involves specifying the prompt template as an argument and utilizing the RetrievalQA.from_chain_type
method aligning the model with the "stuff" chain type and the chosen OpenAI model and retriever object. This setup guarantees that model queries adhere to the template, ensuring consistent and clear interactions. Subsequently, we can focus on addressing specific tasks.
A query string is crafted to pose a particular question, and the run method of the RetrievalQA model is summoned with this query. This step exemplifies the versatility of the RetrievalQA model in managing diverse queries and delivering pertinent answers based on the processed data.
# Create prompt template
prompt_template = """
Use the following pieces of context to answer the question at the end. If
you're not sure, just say so. If there are potential multiple answers,
summarize them as possible answers.
{context}
Question: {question}
Answer:
"""
PROMPT = PromptTemplate(template=prompt_template,
input_variables=["context", "question"])
# Initialize RetrievalQA object
qa_chain = load_qa_chain(OpenAI(), chain_type="stuff")
chain_type_kwargs = {"prompt": PROMPT}
qa = RetrievalQA.from_chain_type(llm=OpenAI(model_name='gpt-4-0613'),
chain_type="stuff",
retriever=docsearch.as_retriever(),
chain_type_kwargs=chain_type_kwargs)
# Query the data
query = "How can I extract tables from a PDF file?"
qa.run(query)
Conclusion
We've walked through the steps to build a Retrieval Augmented Generation system for specific Q+A retrieval. From gathering data to answering queries, each step is crucial to making the system work effectively. With this guide, developers have a clear path to use tools like RAG, Unstructured, SingleStoreDB and LangChain. As technology advances, this knowledge will be a valuable starting point for further exploration and innovation.
Poised to embark on this transformative expedition? Join the vibrant Unstructured community on Slack and sign up for Singlestore Helios for free here.
About Unstructured
Unstructured provides data connectors and transformative engines that adeptly transform unstructured data forms – spanning dense PDFs, dynamic presentations, or images – into the universally recognized JSON format for LLMs and Vector Databases. Its image-to-text model revolutionizes image-based text extraction, endowing users with unparalleled flexibility. Concurrently, its state-of-the-art table extraction capabilities set a new benchmark in data extraction paradigms.